43 python plot with labels
Simple Plot in Python using Matplotlib - GeeksforGeeks Installation of matplotlib library. Step 1: Open command manager (just type "cmd" in your windows start search bar) Step 2: Type the below command in the terminal. cd Desktop. Step 3: Then type the following command. pip install matplotlib. Python Charts - Stacked Bar Charts with Labels in Matplotlib fig, ax = plt.subplots() colors = ['#24b1d1', '#ae24d1'] bottom = np.zeros(len(agg_tips)) for i, col in enumerate(agg_tips.columns): ax.bar(agg_tips.index, agg_tips[col], bottom=bottom, label=col, color=colors[i]) bottom += np.array(agg_tips[col]) ax.set_title('Tips by Day and Gender') ax.legend() Adding Labels to the Bars
python - Adding labels in x y scatter plot with seaborn ... Sep 04, 2017 · I've spent hours on trying to do what I thought was a simple task, which is to add labels onto an XY plot while using seaborn. Here's my code. import seaborn as sns import matplotlib.pyplot as plt %matplotlib inline df_iris=sns.load_dataset("iris") sns.lmplot('sepal_length', # Horizontal axis 'sepal_width', # Vertical axis data=df_iris, # Data source fit_reg=False, # Don't fix a regression ...
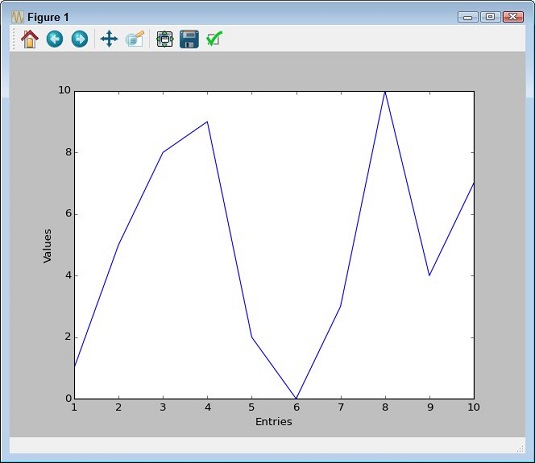
Python plot with labels
Matplotlib.pyplot.xlabels() in Python - GeeksforGeeks The xlabel () function in pyplot module of matplotlib library is used to set the label for the x-axis.. Syntax: matplotlib.pyplot.xlabel (xlabel, fontdict=None, labelpad=None, **kwargs) Parameters: This method accept the following parameters that are described below: xlabel: This parameter is the label text. And contains the string value. How to Add Text Labels to Scatterplot in Python (Matplotlib/Seaborn ... A simple scatter plot can plotted with Goals Scored in x-axis and Goals Conceded in the y-axis as follows. plt.figure (figsize= (8,5)) sns.scatterplot (data=df,x='G',y='GA') plt.title ("Goals Scored vs Conceded- Top 6 Teams") #title plt.xlabel ("Goals Scored") #x label plt.ylabel ("Goals Conceded") #y label plt.show () Basic scatter plot matplotlib - Python plot label - Stack Overflow So in your case to get label = mass you have to use label = "%.1E" % mass with additional formatting options if needed. Most probably you have to rethink your mass variable. To get what you manually typed in the example additionally to a numeric value you need also a string - equivalent to MASS1 unless you put the mass values into an array and ...
Python plot with labels. python - Add x and y labels to a pandas plot - Stack Overflow Apr 06, 2017 · The df.plot() function returns a matplotlib.axes.AxesSubplot object. You can set the labels on that object. ax = df2.plot(lw=2, colormap='jet', marker='.', markersize=10, title='Video streaming dropout by category') ax.set_xlabel("x label") ax.set_ylabel("y label") How to add text labels to a scatterplot in Python? - Data Plot Plus Python Add text labels to Data points in Scatterplot The addition of the labels to each or all data points happens in this line: [plt.text(x=row['avg_income'], y=row['happyScore'], s=row['country']) for k,row in df.iterrows() if 'Europe' in row.region] We are using Python's list comprehensions. Iterating through all rows of the original DataFrame. Graph Plotting in Python | Set 1 - GeeksforGeeks Give a name to x-axis and y-axis using .xlabel () and .ylabel () functions. Give a title to your plot using .title () function. Finally, to view your plot, we use .show () function. Plotting two or more lines on same plot Python import matplotlib.pyplot as plt x1 = [1,2,3] y1 = [2,4,1] plt.plot (x1, y1, label = "line 1") x2 = [1,2,3] y2 = [4,1,3] matplotlib.pyplot.legend — Matplotlib 3.6.0 documentation Note. Specific artists can be excluded from the automatic legend element selection by using a label starting with an underscore, "_". A string starting with an underscore is the default label for all artists, so calling Axes.legend without any arguments and without setting the labels manually will result in no legend being drawn.
Matplotlib Plot A Line (Detailed Guide) - Python Guides You can change the line style in a line chart in python using matplotlib. You need to specify the parameter linestyle in the plot () function of matplotlib. There are several line styles available in python. You can choose any of them. You can either specify the name of the line style or its symbol enclosed in quotes. Python Charts - Pie Charts with Labels in Matplotlib The labels argument should be an iterable of the same length and order of x that gives labels for each pie wedge. For our example, let's say we want to show which sports are most popular at a given school by looking at the number of kids that play each. import matplotlib.pyplot as plt x = [10, 50, 30, 20] labels = ['Surfing', 'Soccer ... Matplotlib Time Series Plot - Python Guides Jan 09, 2022 · Also, read: Matplotlib fill_between – Complete Guide Matplotlib time series scatter plot. Now here we learn to plot time-series graphs using scatter charts in Matplotlib. ... Python Charts - Grouped Bar Charts with Labels in Matplotlib With the grouped bar chart we need to use a numeric axis (you'll see why further below), so we create a simple range of numbers using np.arange to use as our x values. We then use ax.bar () to add bars for the two series we want to plot: jobs for men and jobs for women. fig, ax = plt.subplots(figsize=(12, 8)) # Our x-axis.
Add Labels and Text to Matplotlib Plots: Annotation Examples - queirozf.com Add text to plot; Add labels to line plots; Add labels to bar plots; Add labels to points in scatter plots; Add text to axes; Used matplotlib version 3.x. View all code on this notebook. Add text to plot. See all options you can pass to plt.text here: valid keyword args for plt.txt. Use plt.text(, , ): Plot Pie Chart in Python (Examples) - VedExcel Jun 27, 2021 · Multiple Pie Chart Python. Cool Tip: Learn How to plot stacked area plot in python ! Pie Chart in Python using matplotlib. In this matplotlib pie chart with python example, I will explain you to customize pie chart in python by changing the colors and appearance of the sectors. To plot pie chart in python, use plt.pie() function of matplotlib ... Adding value labels on a Matplotlib Bar Chart - GeeksforGeeks for plotting the data in python we use bar () function provided by matplotlib library in this we can pass our data as a parameter to visualize, but the default chart is drawn on the given data doesn't contain any value labels on each bar of the bar chart, since the default bar chart doesn't contain any value label of each bar of the bar chart it … 7 ways to label a cluster plot in Python — Nikki Marinsek Seaborn makes it incredibly easy to generate a nice looking labeled scatter plot. This style works well if your data points are labeled, but don't really form clusters, or if your labels are long. #plot data with seaborn facet = sns.lmplot(data=data, x='x', y='y', hue='label', fit_reg=False, legend=True, legend_out=True) STYLE 2: COLOR-CODED LEGEND
Python Plot Multiple Lines Using Matplotlib - Python Guides Plot the data (multiple lines) and adding the features you want in the plot (title, color pallete, thickness, labels, annotation, etc…). Show the plot (graph/chart). You can also save the plot. Let's plot a simple graph containing two lines in python. So, open up your IPython shell or Jupiter notebook, and follow the code below:
How To Label The Values Of Plots With Matplotlib Also, as a final touch to the plot I would like to add grid lines as well. This is achieved by calling plt.grid () as seen below. fig, ax = plt.subplots (figsize= (12,8)) plt.plot (x, y) plt.xlabel ("x values", size=12) plt.ylabel ("y values", size=12) plt.title ("Learning more about pyplot with random numbers chart", size=15)
Plot a pie chart in Python using Matplotlib - GeeksforGeeks Nov 30, 2021 · Output: Customizing Pie Chart. A pie chart can be customized on the basis several aspects. The startangle attribute rotates the plot by the specified degrees in counter clockwise direction performed on x-axis of pie chart. shadow attribute accepts boolean value, if its true then shadow will appear below the rim of pie.
Add a title and axis labels to your charts using matplotlib In this post, you will see how to add a title and axis labels to your python charts using matplotlib. If you're new to python and want to get the basics of matplotlib, this online course can be interesting. In the following example, title, x label and y label are added to the barplot using the title (), xlabel (), and ylabel () functions of the ...
Python Matplotlib Implement a Scatter Plot with Labels: A Completed ... First, we will check the length of coord and labels are the same or not by python assert statement. To understand assert statement, you can read this tutorial. Then, we will use plt.scatter (x, y) to draw these scatter points. Finally, we will use plt.annotate () function to display labels of these scatter points. How to use plot_with_labels ...
Matplotlib Bar Chart Labels - Python Guides Here we use the bar () method to plot the bar chart and the ylabel () method to define the y-axis labels. plt.ylabels () "Labels on Y-axis" Read: Matplotlib remove tick labels Matplotlib bar chart tick labels Firstly we have to understand what does tick labels mean. Basically, ticks are the markers and labels is the name given to them.
python - sklearn plot confusion matrix with labels - Stack Overflow You can use the ConfusionMatrixDisplay class within sklearn.metrics directly and bypass the need to pass a classifier to plot_confusion_matrix. It also has the display_labels argument, which allows you to specify the labels displayed in the plot as desired.
python - plot histogram matplotlib with labels on x axis instead of ... import random import matplotlib.pyplot as plt num_families = 10 # set the random seed (for reproducibility) random.seed (42) # setup the plot fig, ax = plt.subplots () # generate some random data x = [random.randint (0, 5) for x in range (num_families)] # create the histogram ax.hist (x, align='left') # `align='left'` is used to center the …
Python Scatter Plot - Python Geeks Scatter plot in Python is one type of a graph plotted by dots in it. The dots in the plot are the data values. To represent a scatter plot, we will use the matplotlib library. To build a scatter plot, we require two sets of data where one set of arrays represents the x axis and the other set of arrays represents the y axis data.
Matplotlib Labels and Title - W3Schools Create Labels for a Plot With Pyplot, you can use the xlabel () and ylabel () functions to set a label for the x- and y-axis. Example Add labels to the x- and y-axis: import numpy as np import matplotlib.pyplot as plt x = np.array ( [80, 85, 90, 95, 100, 105, 110, 115, 120, 125]) y = np.array ( [240, 250, 260, 270, 280, 290, 300, 310, 320, 330])
Python Plotting With Matplotlib (Guide) - Real Python Matplotlib maintains a handy visual reference guide to ColorMaps in its docs. The only real pandas call we're making here is ma.plot (). This calls plt.plot () internally, so to integrate the object-oriented approach, we need to get an explicit reference to the current Axes with ax = plt.gca ().
Matplotlib X-axis Label - Python Guides Use the xlabel () method in matplotlib to add a label to the plot's x-axis. Let's have a look at an example: # Import Library import matplotlib.pyplot as plt # Define Data x = [0, 1, 2, 3, 4] y = [2, 4, 6, 8, 12] # Plotting plt.plot (x, y) # Add x-axis label plt.xlabel ('X-axis Label') # Visualize plt.show ()
Python Scatter Plot - Machine Learning Plus Apr 21, 2020 · Scatter plot is a graph in which the values of two variables are plotted along two axes. It is a most basic type of plot that helps you visualize the relationship between two variables. Concept. What is a Scatter plot? Basic Scatter plot in python; Correlation with Scatter plot; Changing the color of groups of points; Changing the Color and Marker
Plot With Pandas: Python Data Visualization for Beginners Here's how to show the figure in a standard Python shell: >>> >>> import matplotlib.pyplot as plt >>> df.plot(x="Rank", y=["P25th", "Median", "P75th"]) >>> plt.show() Notice that you must first import the pyplot module from Matplotlib before calling plt.show () to display the plot.
Line plot or Line chart in Python with Legends Line 2: Inputs the array to the variable named values Line 3: Plots the line chart with values and choses the x axis range from 1 to 11. Line 4: Displays the resultant line chart in python. So the output will be Multiple Line chart in Python with legends and Labels: lets take an example of sale of units in 2016 and 2017 to demonstrate line ...
How to Add Labels in a Plot using Python? - GeeksforGeeks By using pyplot () function of library we can add xlabel () and ylabel () to set x and y labels. Example: Let's add Label in the above Plot. Python. # python program for plots with label. import matplotlib. import matplotlib.pyplot as plt. import numpy as np. # Number of children it was default in earlier case.
matplotlib - Python plot label - Stack Overflow So in your case to get label = mass you have to use label = "%.1E" % mass with additional formatting options if needed. Most probably you have to rethink your mass variable. To get what you manually typed in the example additionally to a numeric value you need also a string - equivalent to MASS1 unless you put the mass values into an array and ...
How to Add Text Labels to Scatterplot in Python (Matplotlib/Seaborn ... A simple scatter plot can plotted with Goals Scored in x-axis and Goals Conceded in the y-axis as follows. plt.figure (figsize= (8,5)) sns.scatterplot (data=df,x='G',y='GA') plt.title ("Goals Scored vs Conceded- Top 6 Teams") #title plt.xlabel ("Goals Scored") #x label plt.ylabel ("Goals Conceded") #y label plt.show () Basic scatter plot
Matplotlib.pyplot.xlabels() in Python - GeeksforGeeks The xlabel () function in pyplot module of matplotlib library is used to set the label for the x-axis.. Syntax: matplotlib.pyplot.xlabel (xlabel, fontdict=None, labelpad=None, **kwargs) Parameters: This method accept the following parameters that are described below: xlabel: This parameter is the label text. And contains the string value.
Post a Comment for "43 python plot with labels"